In the world of PHP programming, understanding variable scope is crucial for writing efficient and effective code. As a developer, you may often encounter situations where you need to access a variable defined within a function from outside that function. This can lead to confusion, especially for those who are new to PHP programming. However, grasping the concept of variable scope will not only enhance your coding skills but also improve the overall performance of your applications.
In PHP, variables have different scopes, which determine where they can be accessed within your code. The two main types of variable scopes in PHP are global and local. Local variables are those defined within a function, while global variables are accessible throughout the script. Understanding how to effectively use global variables and manipulate them can open up a whole new world of possibilities for your projects.
This article will delve into the intricacies of using a variable outside of a function in PHP, providing you with a comprehensive guide on the best practices and common pitfalls. Whether you are a novice or an experienced developer, this guide will help you navigate the complex waters of variable scope in PHP.
What is Variable Scope in PHP?
Variable scope refers to the visibility and lifespan of a variable within a program. In PHP, there are primarily two scopes to be aware of:
- Local Scope: Variables declared within a function are local to that function and cannot be accessed outside of it.
- Global Scope: Variables declared outside of functions can be accessed throughout the script, including inside functions, if properly referenced.
How to Declare a Global Variable in PHP?
To use a variable outside of a function in PHP, you must declare it as a global variable. This can be achieved using the global
keyword. Here’s a simple example:
$globalVar ="I am a global variable."; function myFunction() { global $globalVar; // Accessing the global variable echo $globalVar; } myFunction(); // Outputs: I am a global variable.
Why Use Global Variables?
Global variables can be useful in various scenarios, such as:
- When you need to maintain state across multiple function calls.
- For configuration settings that should be accessible throughout the application.
- When sharing data between different parts of your code without passing them as function parameters.
Can I Access a Local Variable Outside of Its Function?
No, local variables cannot be accessed outside of the function in which they are declared. If you try to access a local variable from outside its function, you will encounter an "undefined variable" error. This is a fundamental aspect of variable scope in PHP.
How to Pass Variables to Functions?
If you need to use data within a function without declaring it as global, you can pass variables as parameters. Here’s how:
function myFunction($param) { echo $param; } $myVar ="Hello, World!"; myFunction($myVar); // Outputs: Hello, World!
What Are the Common Pitfalls of Using Global Variables?
While global variables can be convenient, they come with their own set of challenges:
- Unintended Modifications: Since global variables can be changed from anywhere in the code, it may lead to unexpected behaviors and bugs.
- Code Maintainability: Overuse of global variables can make your code harder to read and maintain.
- Namespace Pollution: Using many global variables can clutter the global namespace and lead to conflicts.
How to Ensure Safe Use of Global Variables?
To minimize issues while using global variables, consider the following best practices:
- Limit the number of global variables in your code.
- Use meaningful names to avoid conflicts.
- Document the purpose of each global variable clearly.
Can I Use Static Variables to Maintain State?
Yes, static variables can be an excellent alternative to global variables when you want to maintain state between function calls without polluting the global namespace. Static variables retain their value even after the function has finished executing.
function myFunction() { static $count = 0; // Static variable $count++; echo $count; } myFunction(); // Outputs: 1 myFunction(); // Outputs: 2
Conclusion: Mastering Variable Scope in PHP
Understanding how to use a variable outside of a function in PHP is a fundamental skill that every developer should master. By leveraging global variables appropriately and knowing the limitations of local variables, you can write cleaner and more efficient code. Remember to follow best practices to avoid common pitfalls associated with global variables.
As you continue to develop your PHP skills, keep experimenting with variable scope and explore different ways to manage state and data within your applications. The more you practice, the more confident you will become in handling variables effectively!
Article Recommendations
- How Old Is Helena Vestergaard
- Kelsey Lawrence And Dabb Fan Bus Video
- Jerry Lorenzo Dad
- Nikocado Avocado Fit
- What Is Tortured Poets
- Josh Gates Dating Now
- Percy Jackson Logan Lerman
- How Old Was Michael J Fox Back To The Future
- Good Morning Quote For A Friend
- Glenn Close Michael Douglas
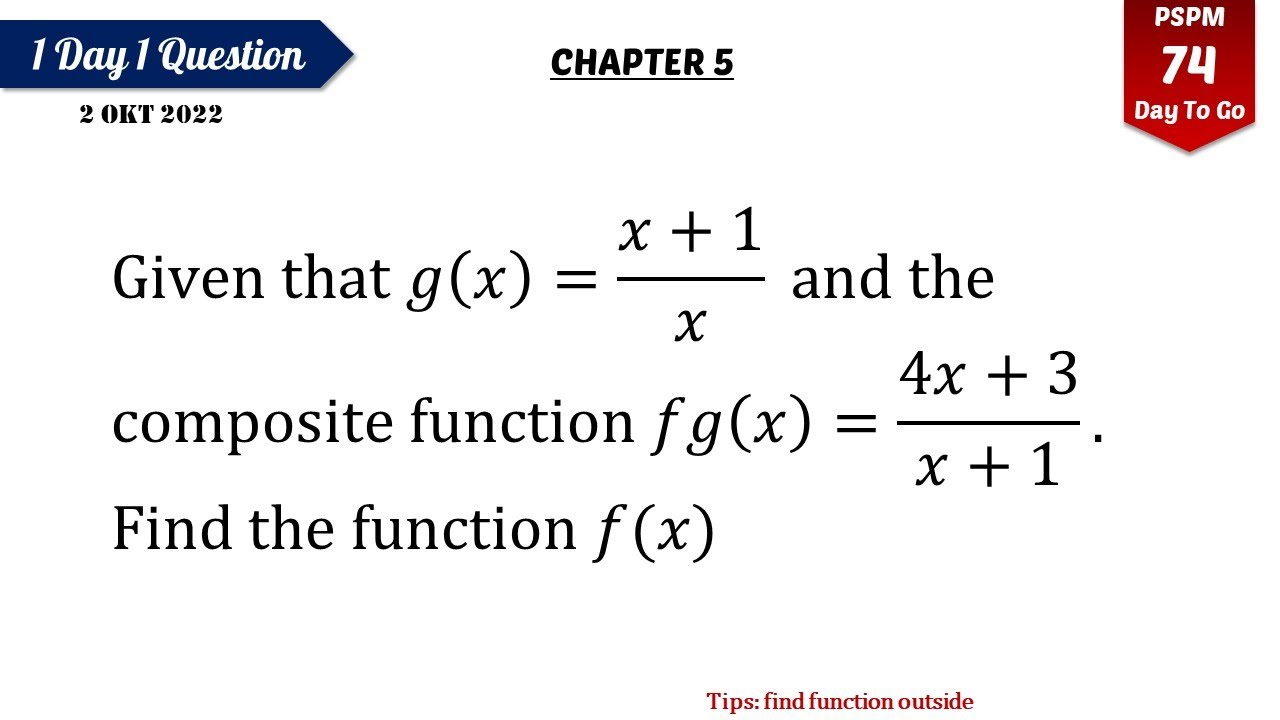
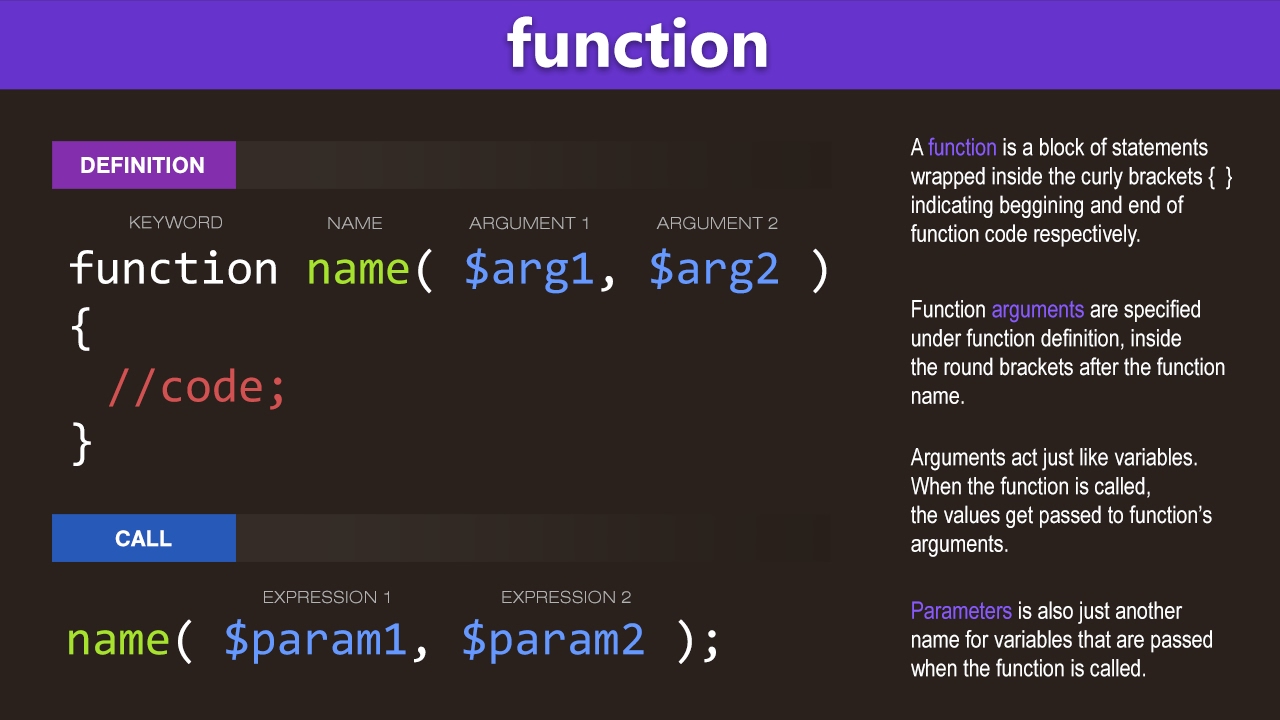
