The Go programming language, commonly known as Golang, has gained significant popularity among developers for its simplicity and efficiency. One of the core features that makes Go stand out is its use of interfaces, which allow for a flexible and powerful way to define behavior in your programs. In this article, we will explore the concept of Golang interface to struct, delving into its syntax, practical applications, and best practices.
Interfaces in Go provide a way to specify a contract that types must adhere to, promoting loose coupling and greater code reusability. This article will guide you through the intricacies of using interfaces with structs, offering insights into how they can enhance your Go applications. By the end of this article, you'll have a solid understanding of how to effectively use interfaces within your Go code.
Whether you're a seasoned Go developer or just starting your journey with the language, mastering interfaces is essential for writing clean and maintainable code. Let's dive deep into the world of Golang interface to struct, examining the syntax, real-world use cases, and expert tips to help you become proficient in this powerful feature.
Table of Contents
- Understanding Interfaces in Go
- Defining an Interface
- Implementing an Interface with Structs
- Example of Interfaces with Structs
- Advantages of Using Interfaces
- Best Practices for Working with Interfaces
- Common Mistakes to Avoid
- Conclusion
Understanding Interfaces in Go
In Go, an interface is a type that specifies a contract of methods that a struct can implement. It allows you to define a set of behaviors that can be shared across different types without explicitly specifying how those behaviors are implemented. This decoupling of behavior and implementation is one of the key advantages of using interfaces.
For example, consider an interface named Shape
that defines a method Area
. Any struct that implements this method can be considered a Shape
, regardless of its specific type.
Interface Syntax
The syntax for defining an interface in Go is straightforward:
type InterfaceName interface { MethodName(parameters) returnType }
Defining an Interface
To define an interface in Go, you use the type
keyword followed by the interface name and the method signatures it should contain. Here's a simple example:
type Shape interface { Area() float64 }
In this example, any struct that wants to be considered a Shape
must implement the Area
method, which returns a float64 value representing the area of the shape.
Implementing an Interface with Structs
Once you have defined an interface, you can implement it in any struct. There is no need for explicit declaration of intent to implement an interface; if the struct has the required methods, it satisfies the interface. Here's how you can do it:
type Rectangle struct { Width float64 Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height }
In this example, we have defined a Rectangle
struct and implemented the Area
method, thereby making Rectangle
a Shape
.
Example of Interfaces with Structs
Let’s look at a more comprehensive example that includes multiple shapes implementing the same interface:
type Circle struct { Radius float64 } func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } func PrintArea(s Shape) { fmt.Printf("Area: %f\n", s.Area()) }
In this example, both Rectangle
and Circle
implement the Shape
interface. We can use the PrintArea
function to print the area of any shape that implements the Shape
interface:
r := Rectangle{Width: 3, Height: 4} c := Circle{Radius: 5} PrintArea(r) // Area: 12.000000 PrintArea(c) // Area: 78.539816
Advantages of Using Interfaces
Using interfaces in your Go programs offers several advantages:
- Decoupling: Interfaces help decouple your code, allowing for easier testing and maintenance.
- Flexibility: You can pass different types that implement the same interface, making your functions more flexible.
- Reusability: You can reuse the same function for different types without modifying the function itself.
- Abstraction: Interfaces provide a level of abstraction, allowing you to focus on what a type does rather than how it does it.
Best Practices for Working with Interfaces
To effectively utilize interfaces in your Go applications, consider the following best practices:
- Keep interfaces small: Aim for small, focused interfaces to promote better design and easier implementation.
- Use interface types as parameters: When designing functions, use interface types for flexibility and to allow for any type that satisfies the interface.
- Avoid unnecessary interfaces: Don’t create interfaces for types that are not intended to be reused or implemented by multiple types.
Common Mistakes to Avoid
When working with interfaces, be mindful of the following common mistakes:
- Ignoring interface satisfaction: Remember that a type satisfies an interface simply by implementing its methods, even if it doesn't explicitly declare it.
- Overcomplicating interfaces: Avoid creating overly complex interfaces that are difficult to implement and understand.
- Not using interfaces: Don’t shy away from using interfaces; they are a powerful feature that can greatly enhance your code.
Conclusion
In this article, we explored the concept of Golang interface to struct, detailing its syntax, implementation, and practical advantages. By understanding how to define and implement interfaces, you can write more flexible, maintainable, and reusable code in Go. We encourage you to practice these concepts in your projects to fully grasp their potential.
If you found this article helpful, please leave a comment below, share it with your fellow developers, or check out our other articles for more insights into Golang and programming best practices.
Final Thoughts
Thank you for taking the time to read this article! We hope to see you back here for more quality content. Happy coding!
Article Recommendations
- Simon Cowellsons Name
- The Prince Of Denmark
- John Krasinski Weight
- Is Taylor Swift Died
- Evangeline Lilly Parents
- What Is Tortured Poets
- Where Is The Swans Streaming
- Identify The Two Longest Rivers In The U S
- Nigerian Actors Hollywood
- Aj And Kandi Burruss


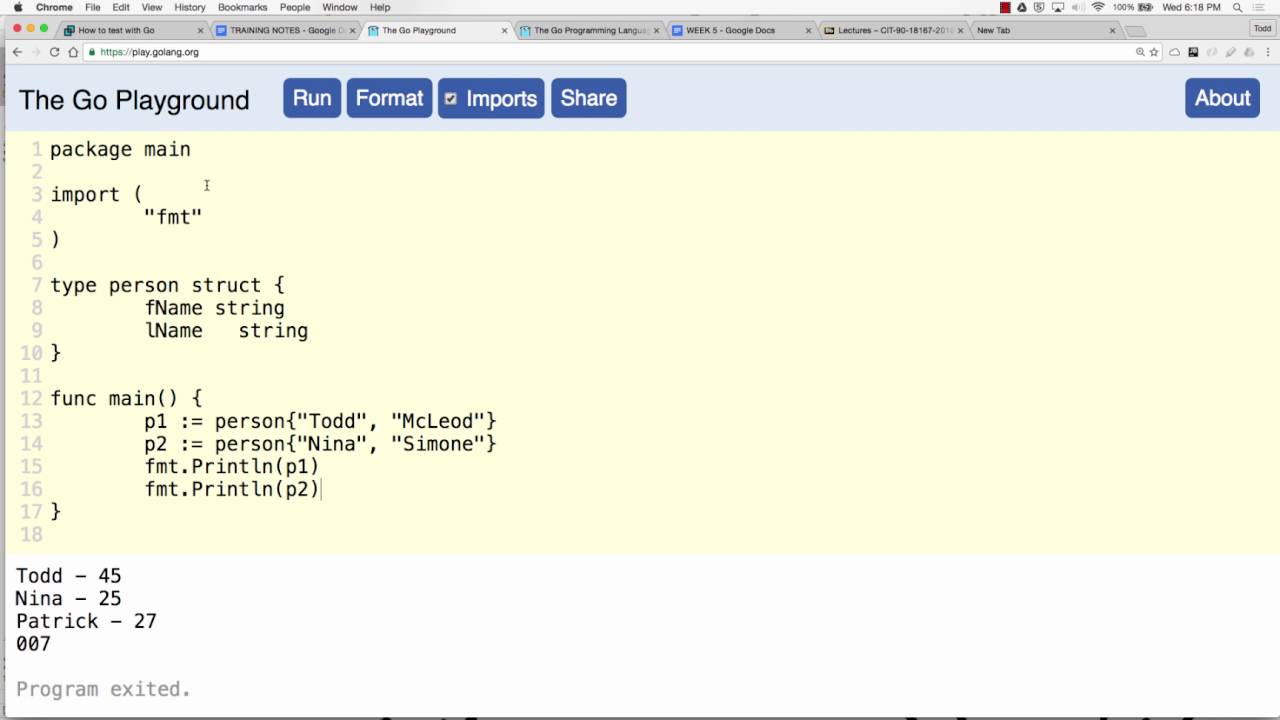