Programming languages are filled with a variety of rules and expectations that can sometimes lead to confusion for developers. One such common error encountered is the message "expected a type specifier." This error typically arises when the compiler or interpreter anticipates a specific type of data but instead finds something that doesn't fit the expected mold. This article aims to shed light on this error, its implications, and how to effectively troubleshoot it.
The "expected a type specifier" error can occur in numerous programming languages, including C, C++, and Java, among others. This message signifies that the code is lacking a clear declaration of a type, which is essential for proper compilation. Understanding the reasons behind this error can save programmers a significant amount of time, allowing them to write more efficient and error-free code. Furthermore, mastering this aspect of coding can enhance one’s overall programming skills.
As we delve deeper into the nuances of the "expected a type specifier" error, we will cover various aspects such as common scenarios where this error arises, ways to prevent it, and best practices for debugging. Whether you're a novice programmer or an experienced developer, grasping the importance of type specifiers will undoubtedly improve your coding journey.
What is a Type Specifier?
A type specifier is a keyword in programming that defines the type of data a variable can hold. For instance, in C++, you might use keywords like int
, float
, or char
as type specifiers. These keywords inform the compiler about the kind of data that will be stored in the variable, which in turn helps in memory management and data integrity.
Why Do We Need Type Specifiers?
Type specifiers play a crucial role in ensuring that the data being handled is of the correct type. This helps prevent bugs and errors that could arise from type mismatches. For example, attempting to perform mathematical operations on a string instead of a number can lead to unexpected results. Therefore, having a clear type specifier allows the compiler to perform type-checking at compile-time, which enhances program reliability.
What Causes the "Expected a Type Specifier" Error?
There are several reasons why a programmer might encounter the "expected a type specifier" error. Some of the most common causes include:
- Forgetting to declare a variable's type.
- Misspelling a type specifier.
- Using a type specifier that is not defined in the given context.
- Incorrectly structuring code that leads to ambiguity in type declaration.
How Can You Fix the "Expected a Type Specifier" Error?
Fixing the "expected a type specifier" error involves a careful review of your code. Here are some steps that can help resolve this issue:
- Check for missing type declarations for your variables.
- Ensure that all type specifiers are spelled correctly.
- Make sure the type you're trying to use is valid and defined in your programming environment.
- Review your code structure to avoid ambiguity in type declarations.
Can You Provide an Example?
Certainly! Let's consider a simple example in C++:
#include int main() { int number; // Correct type specifier float pi; // Correct type specifier unknownType variable; // This will throw an error return 0; }
In the above example, the line unknownType variable;
would trigger the "expected a type specifier" error because unknownType
is not a defined type in C++. Ensuring that all variable types are correctly specified can prevent this error.
Are There Tools to Help Identify This Error?
Yes, there are several tools and IDEs (Integrated Development Environments) that can help identify such errors. Some popular ones include:
- Visual Studio
- Code::Blocks
- Eclipse
- JetBrains IntelliJ IDEA
These tools often provide real-time error detection, which can help you catch the "expected a type specifier" error before compiling your code.
Conclusion
In conclusion, understanding the "expected a type specifier" error is essential for any programmer. By familiarizing yourself with type specifiers, their significance, and common pitfalls, you can enhance your coding proficiency. Remember that careful attention to detail in your code can prevent such errors and lead to smoother programming experiences. As you continue your programming journey, bear in mind the importance of type declarations, and always strive for clarity in your code.
Article Recommendations
- How Many Seasons Is Steve On Shameless
- Younger Barbara Bush
- Who Plays Ally In Austin And Ally
- What Former Presidents Are Still Alive
- Jason Momoa Amber Heard
- Carta Astral Donal Trump
- Cnn What Does Donal Trump Want To Do With Violence
- Ryan Paevey Birthday
- Is Taylor Swift Died
- Great British Bake Off Shop


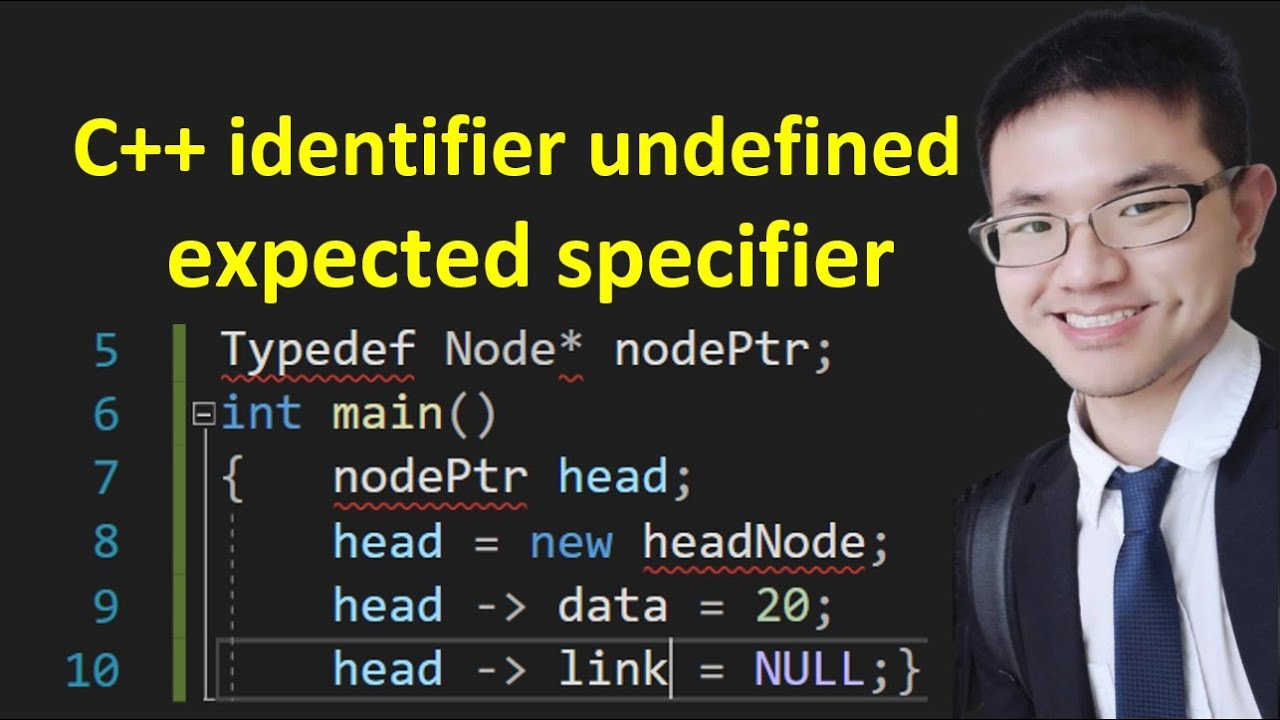